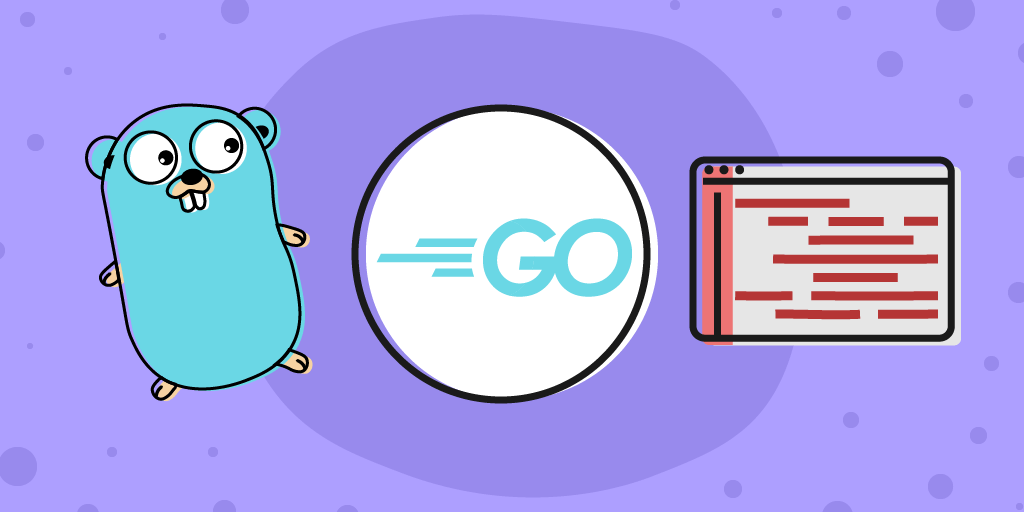
Testing is an essential aspect of software development. In Go (Golang), writing tests is both straightforward and highly encouraged. Go’s built-in testing framework makes it easy to create and run tests, ensuring that your code functions as intended and continues to work correctly as it evolves. In this blog, we’ll explore how to write tests in Go, including best practices and code snippets to illustrate the concepts.
Creating Test Files
To start writing tests in Go, create a separate test file for each package you want to test. Test files should have a name that ends with _test.go
, indicating that they contain test code. For example, if your package is named myapp
, the corresponding test file should be named myapp_test.go
.
Writing Test Functions
Test functions in Go follow a specific naming convention: they begin with Test
and then include the name of the function they are testing. For instance, if you have a function named Add
in your package, the corresponding test function should be named TestAdd
.
Here’s an example of a simple test function:
// In file: math.go
package mymath
func Add(a, b int) int {
return a + b
}
// In file: math_test.go
package mymath
import "testing"
func TestAdd(t *testing.T) {
result := Add(2, 3)
expected := 5
if result != expected {
t.Errorf("Add(2, 3) = %d; expected %d", result, expected)
}
}
Running Tests
To run tests in Go, use the go test
command followed by the package name. Go will automatically discover and execute all test functions in the package.
$ go test mymath
Testing Framework and Assertions
Go’s testing framework provides a set of helper functions, including t.Errorf
, t.Fail
, and t.FailNow
, to assist in writing tests and generating test output. These functions are used within test functions to provide feedback about the test’s success or failure.
Table-Driven Tests
Table-driven tests are a powerful testing pattern in Go. They involve creating a data structure (usually a slice or a map) to define a set of input/output pairs for a specific function. The test function then iterates over the data structure, calling the function with each input and verifying the output.
// In file: math_test.go
package mymath
import "testing"
func TestAdd(t *testing.T) {
tests := []struct {
a, b, expected int
}{
{2, 3, 5},
{-1, 1, 0},
{0, 0, 0},
}
for _, test := range tests {
result := Add(test.a, test.b)
if result != test.expected {
t.Errorf("Add(%d, %d) = %d; expected %d", test.a, test.b, result, test.expected)
}
}
}
Benchmarking
In addition to writing tests, Go also supports benchmarking. Benchmark functions follow a similar naming convention as test functions: they start with Benchmark
and then include the name of the function they are benchmarking.
Here’s an example of a simple benchmark function:
// In file: math_test.go
package mymath
import "testing"
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
_ = Add(2, 3)
}
}
Running Benchmarks
To run benchmarks in Go, use the go test
command with the -bench
flag followed by the package name.
$ go test -bench mymath
Handling Errors in Tests
When writing tests in Go, it’s essential to consider error scenarios as well. Properly handling errors ensures that your code behaves gracefully when unexpected situations arise. Go provides the testing.T
type’s Error
, Fail
, and Fatal
methods to handle errors in tests.
Here’s an example of testing a function that returns an error:
// In file: fileutil.go
package myutil
import (
"os"
)
func OpenFile(filename string) (*os.File, error) {
file, err := os.Open(filename)
if err != nil {
return nil, err
}
return file, nil
}
// In file: fileutil_test.go
package myutil
import "testing"
func TestOpenFile(t *testing.T) {
_, err := OpenFile("nonexistentfile.txt")
if err == nil {
t.Error("Expected error but got nil")
}
}
Subtests and Test Helper Functions
Go also allows you to create subtests and test helper functions to organize and modularize your test code. Subtests allow you to group related tests together, and test helper functions can be used to avoid code duplication and enhance test readability.
Here’s an example of using subtests and test helper functions:
// In file: math_test.go
package mymath
import "testing"
func TestAdd(t *testing.T) {
tests := []struct {
a, b, expected int
}{
{2, 3, 5},
{-1, 1, 0},
{0, 0, 0},
}
for _, test := range tests {
t.Run(fmt.Sprintf("%d+%d", test.a, test.b), func(t *testing.T) {
result := Add(test.a, test.b)
if result != test.expected {
t.Errorf("Add(%d, %d) = %d; expected %d", test.a, test.b, result, test.expected)
}
})
}
}
Test Coverage
Test coverage is a metric that indicates how much of your code is covered by tests. It helps identify untested code paths and potential vulnerabilities. To get test coverage, use the -cover
flag with the go test
command:
$ go test -cover mymath
Continuous Integration and Testing
Integrating your test suite into a Continuous Integration (CI) pipeline is crucial for maintaining code quality and catching issues early in the development process. Popular CI tools like Jenkins, CircleCI, and GitHub Actions can automatically run your tests whenever you push changes to your repository.
Conclusion
Writing tests in Go is not only simple but also a critical practice to ensure your code’s correctness and reliability. By organizing tests into separate test files, creating test functions with meaningful names, and using table-driven tests, you can efficiently write thorough and maintainable test suites. Additionally, benchmarking allows you to identify performance bottlenecks and optimize critical parts of your code.
Remember that writing tests is an ongoing process as your code evolves. Regularly run your tests and maintain high test coverage to keep your Go projects robust and bug-free. Embrace the testing culture, and you’ll find that it leads to cleaner, more reliable, and better-performing code. Happy testing!