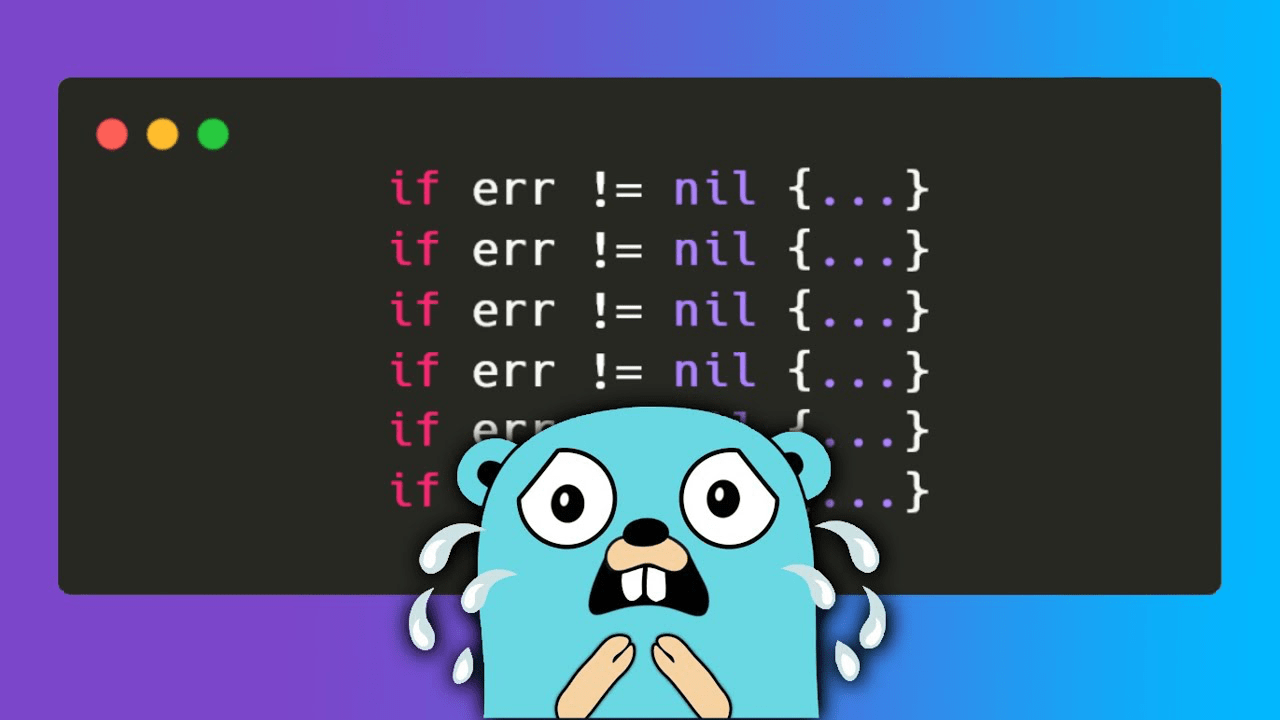
Introduction
Error handling is a critical aspect of software development. In Go (Golang), it plays a pivotal role in ensuring the robustness, reliability, and maintainability of applications. Unlike some other languages, Go advocates for explicit error handling through the use of the error
interface, making it easy to identify and address potential issues in code. In this comprehensive guide, we’ll delve into the principles and best practices of error handling in Go, including error types, returning errors, checking errors, using error codes, handling multiple errors, and panic and recovery mechanisms.
Error Type in Go
In Go, an error is represented by the error
interface, which consists of a single method:
type error interface {
Error() string
}
When a function can encounter an error, it usually returns both a result and an error. By convention, the last return value is the error, and it is set to nil
when the function completes successfully. If an error occurs, the error value contains information about the problem.
Returning Errors
Go’s idiomatic error handling involves returning errors alongside results from functions. This explicit approach ensures that the caller must handle the error, reducing the chances of overlooking potential issues.
Here’s an example of a function that divides two numbers and returns an error if the divisor is zero:
func Divide(a, b float64) (float64, error) {
if b == 0 {
return 0, fmt.Errorf("cannot divide by zero")
}
return a / b, nil
}
Checking Errors
When calling functions that return errors, it’s crucial to check the error value. Ignoring errors can lead to unexpected behavior and bugs. You can check the error using a simple if
statement or the errors.Is
function for more complex scenarios.
result, err := Divide(10, 2)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
Error Codes
In some situations, providing numeric error codes along with error messages can be helpful for programmatically identifying specific errors. To incorporate error codes, you can define a custom error type that includes an additional field for the error code.
Here’s an example of a custom error type with an error code:
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error %d: %s", e.Code, e.Message)
}
By using custom error types with error codes, you can easily differentiate between different types of errors and take appropriate actions based on the error code.
Handling Multiple Errors
In real-world scenarios, functions may execute multiple operations that can return errors. When dealing with multiple errors, you can aggregate them using the multierror
package or define your own error type to hold multiple errors.
Here’s an example of using the multierror
package:
errs := &multierror.Error{}
err1 := SomeOperation()
err2 := AnotherOperation()
errs = multierror.Append(errs, err1, err2)
if errs.ErrorOrNil() != nil {
fmt.Println("Errors occurred:", errs.Error())
}
Panic and Recover
In exceptional situations where the program cannot proceed safely, you can use the panic
function to terminate the program abruptly. However, it’s essential to use recover
it to handle panics gracefully and prevent a complete program crash.
Conclusion
Error handling in Go is a fundamental practice that ensures the reliability and stability of your applications. By following the conventions and best practices outlined in this comprehensive guide, you can create clean and robust error-handling code that enhances the user experience and facilitates debugging.
Remember, error handling is not a one-size-fits-all approach. Adapt your error-handling strategy based on the specific requirements of your application and the context in which it operates. Embrace the art of error handling in Go, including the use of error codes when appropriate, and your code will be more resilient and maintainable, leading to a better overall developer experience and happier users. Happy coding!